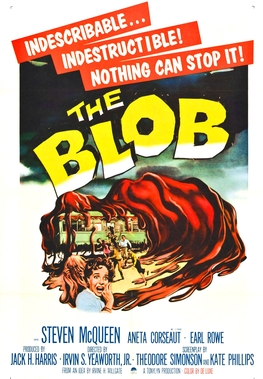
https://en.wikipedia.org/wiki/The_Blob
Comparing File and Blob Storage in AWS, GCP, and Azure
Introduction
Cloud storage is one of the foundational services of cloud computing, providing scalable, reliable, and cost-effective solutions for storing and managing data.
AWS, GCP, and Azure each offer file and blob storage solutions, but they have unique features, pricing models, and integrations.
What Are File and Blob Storage?
File Storage
File storage is a hierarchical storage system that organizes data into directories and files. It is useful for applications that require shared storage, such as network file systems (NFS) and SMB.
Blob Storage
Blob (Binary Large Object) storage is designed for unstructured data such as images, videos, backups, and logs. It is optimized for high durability, scalability, and low-cost storage.
Cloud Storage Solutions
AWS Storage Solutions
- Amazon S3 (Simple Storage Service): AWS’s blob storage solution, designed for high availability and durability.
- Amazon EFS (Elastic File System): A managed file storage service that supports the NFS protocol.
- Amazon FSx: A fully managed file storage solution supporting Windows File Server and Lustre.
Google Cloud Storage Solutions
- Google Cloud Storage: GCP’s object storage solution, similar to Amazon S3.
- Filestore: A fully managed file storage service that supports NFS.
- Persistent Disks: Block storage that can be used similarly to file storage for VMs.
Azure Storage Solutions
- Azure Blob Storage: Microsoft’s object storage solution, similar to Amazon S3.
- Azure Files: A fully managed file share supporting SMB and NFS.
- Azure NetApp Files: A high-performance file storage service for enterprise applications.
Key Differences
Feature | AWS (S3, EFS, FSx) | GCP (Cloud Storage, Filestore) | Azure (Blob Storage, Files) |
---|
Blob Storage | Amazon S3 | Google Cloud Storage | Azure Blob Storage |
File Storage | EFS (NFS), FSx (Windows, Lustre) | Filestore (NFS) | Azure Files (SMB, NFS) |
Durability | 99.999999999% (11 9s) | 99.999999999% (11 9s) | 99.999999999% (11 9s) |
Availability | 99.99% (S3 Standard) | 99.95% | 99.99% |
Cold Storage | Glacier | Archive Storage | Cool/Archive Tiers |
Regional Availability | Global | Global | Global |
Protocol Support | S3 API, NFS, SMB | GCS API, NFS | REST API, SMB, NFS |
Common Problems They Solve
- Scalable storage for large datasets
- Data backup and disaster recovery
- Hosting static websites (S3, Cloud Storage, Blob Storage)
- File sharing across multiple applications and users
- Storing logs, images, and media content
Code Samples
AWS S3
Python (Boto3)
1
2
3
4
| import boto3
s3 = boto3.client('s3')
s3.upload_file('localfile.txt', 'my-bucket', 'remote-file.txt')
|
C# (AWS SDK)
1
2
3
4
5
6
| using Amazon.S3;
using Amazon.S3.Transfer;
var s3Client = new AmazonS3Client();
var transferUtility = new TransferUtility(s3Client);
await transferUtility.UploadAsync("localfile.txt", "my-bucket");
|
Google Cloud Storage
Python
1
2
3
4
5
6
| from google.cloud import storage
client = storage.Client()
bucket = client.bucket('my-bucket')
blob = bucket.blob('remote-file.txt')
blob.upload_from_filename('localfile.txt')
|
C#
1
2
3
4
5
6
7
8
9
| using Google.Cloud.Storage.V1;
var storageClient = StorageClient.Create();
var bucketName = "my-bucket";
var localPath = "localfile.txt";
var objectName = "remote-file.txt";
using var fileStream = File.OpenRead(localPath);
storageClient.UploadObject(bucketName, objectName, null, fileStream);
|
Azure Blob Storage
Python
1
2
3
4
5
6
7
8
| from azure.storage.blob import BlobServiceClient
blob_service_client = BlobServiceClient.from_connection_string("your_connection_string")
container_client = blob_service_client.get_container_client("my-container")
blob_client = container_client.get_blob_client("remote-file.txt")
with open("localfile.txt", "rb") as data:
blob_client.upload_blob(data)
|
C#
1
2
3
4
5
6
7
8
9
| using Azure.Storage.Blobs;
var blobServiceClient = new BlobServiceClient("your_connection_string");
var blobContainerClient = blobServiceClient.GetBlobContainerClient("my-container");
var blobClient = blobContainerClient.GetBlobClient("remote-file.txt");
using FileStream uploadFileStream = File.OpenRead("localfile.txt");
await blobClient.UploadAsync(uploadFileStream);
uploadFileStream.Close();
|
Key Ideas Table
Concept | Explanation |
---|
Amazon S3 | AWS’s scalable blob storage solution |
Google Cloud Storage | GCP’s counterpart to S3 |
Azure Blob Storage | Microsoft’s object storage alternative |
File Storage | Hierarchical storage for shared access |
Blob Storage | Optimized for unstructured data |
Cold Storage | Cost-effective storage for rarely accessed data |
References